728x90
1. Command Pattern 이란 무엇인가요?
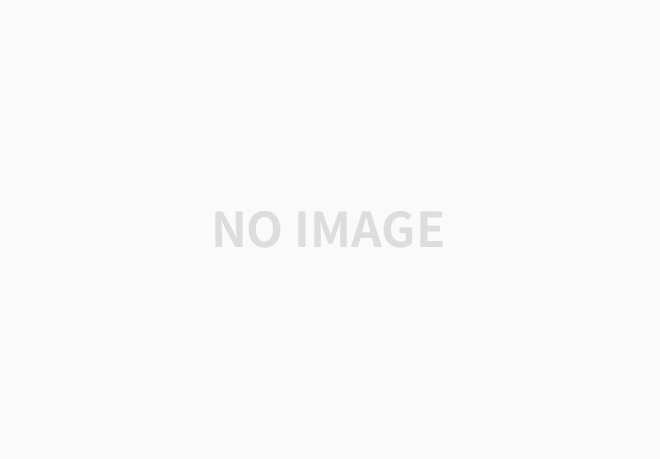
- 이벤트가 발생했을 때 실행될 기능이 다양하면서도 변경이 필요한 경우
- 1번 버튼을 누르면 재생이 되고, 2번 버튼을 누르면 정지가 됨
- 실행될 기능을 캠슐화함
- 기능의 실행을 요구하는 invoker, 클래스와 실제 기능을 실행하는 receiver 클래스 사이의 의존성 제거함
- 실행될 기능의 변경에도 invoker 클래스를 변경하지 않아도 사용할 수 있음
2. Command Pattern을 사용함으로써 어떤 장점이 있을까요?
- 기존 클래스가 변경되지 않으면서 명령을 쉽게 추가할 수 있음
- 실제로 작업을 수행하는 개체에서 작업을 호출하는 개체를 분리함
- 이벤트를 발생시키는 클래스를 변경하지 않고 새 명령을 추가할 수 있으므로 확장성 좋음
- 즉, 버튼을 누를 때 버튼 클래스를 변경하지 않아도 새 명령을 추가할 수 있음
3. Command Pattern을 사용함으로써 어떤 단점이 있을까요?
- 개별 명령에 따라 클래스를 만들어야하므로, 명령이 많아지면 클래스도 많아짐
4. Command Pattern을 언제 사용할까요?
- 작업에 따라 개체를 parameterize해야할 때
- 다른 시간에 요청을 생성하고 실행해야 하는 경우
- When you need to create and execute requests at different times.
- 롤백, 로깅, 트랜잭션 기능을 지원해야 하는 경우
- 아래의 Invoker를 보면 List로 저장되있음
- List에는 무엇을 실행했는지 기록되어있음
- 명령들을 기록해놓음 → logging
- 기록했기 때문에 취소, 되돌리기, 한번에 실행할 수 있음 → rollback, transaction
5. Java api에서 Command Pattern을 사용한 예가 있을까요?
- Runnable
<code />
public class Main {
public static void main(String[] args) {
Thread t1 = new Thread(new Command());
t1.start();
}
}
class Command implements Runnable{
@Override
public void run() {
System.out.println("Hi");
}
}
- javax.swing.Action
6. Example Code(Baeldung)
6.1. Client
<code />
public class Client {
public static void main(String[]args) {
TextFileOpertionExecutor textFileOpertionExecutor
= new TextFileOpertionExecutor();
System.out.println(textFileOpertionExecutor.executeOperation(
new OpenTextFileOperation(new TextFile("file1.txt"))));
System.out.println(textFileOpertionExecutor.executeOperation(
new SaveTextFileOperation(new TextFile("file2.txt"))
));
}
}
6.2. Invoker
<code />
//invoker
public class TextFileOpertionExecutor {
private final List<TextFileOperation> textFileOperations
= new ArrayList<>();
public String executeOperation(TextFileOperationtextFileOperation) {
textFileOperations.add(textFileOperation);
returntextFileOperation.execute();
}
}
6.3. Receiver
<code />
//receiver
public class TextFile {
private String name;
public TextFile(Stringname) {
this.name =name;
}
public String open() {
return "Opening file " + name;
}
public String save() {
return "Saving file " + name;
}
}
6.4. Command
<code />
//Command
@FunctionalInterface
public interface TextFileOperation {
String execute();
}
6.5. Concrete Class
<code />
//Concrete class
public class OpenTextFileOperation implements TextFileOperation {
private TextFile textFile;
public OpenTextFileOperation(TextFile textFile) {
this.textFile = textFile;
}
@Override
public String execute() {
return textFile.open();
}
}
//Concrete class
public class SaveTextFileOperation implements TextFileOperation{
private TextFile textFile;
public SaveTextFileOperation(TextFile textFile) {
this.textFile = textFile;
}
@Override
public String execute() {
return textFile.save();
}
}
7. REFERENCES
728x90
'Design Pattern' 카테고리의 다른 글
Decorator Pattern (0) | 2022.11.06 |
---|---|
Template Callback Pattern (0) | 2022.11.01 |
Singleton Pattern (0) | 2022.03.17 |
State Pattern (0) | 2022.03.12 |
Strategy Pattern (0) | 2022.03.04 |